Basic Theory
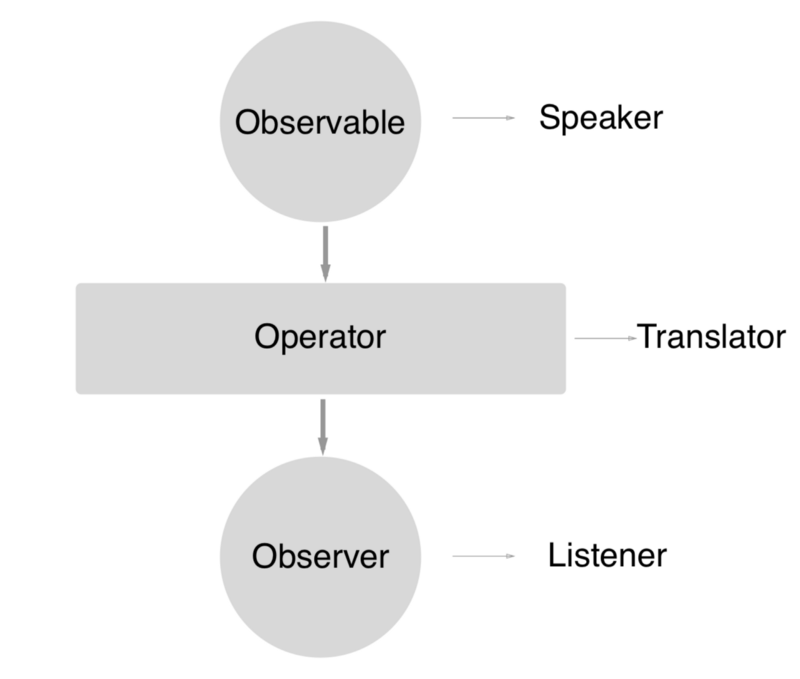
- Flowable: 多个流,响应式流和背压
- Observable: 多个流,无背压
- Single: 只有一个元素或者错误的流
Testing Model
1 | class DataManager(size: Int = 16) { |
Transforming Observables
Map
transform the items emitted by an Observable by applying a function to each item

Example 1
1 | Observable.just(1, 2, 3, 4, 5) |
flatMap vs concatMap
transform the items emitted by an Observable into Observables, then flatten the emissions from those into a single Observable
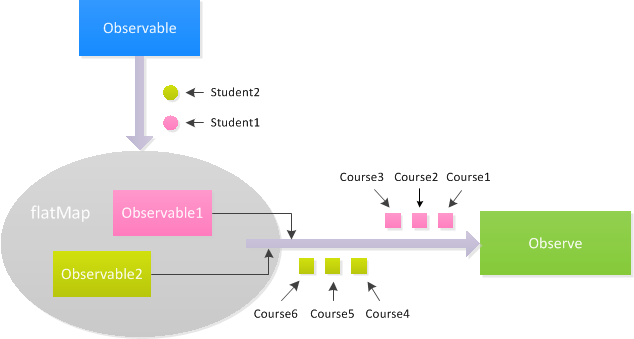
Example 1
1 | val dataManager = DataManager() |
1 | origin = [Student(name=Jack-0, courses=[Course(name=English-0)]), Student(name=Jack-1, courses=[Course(name=English-1)]), Student(name=Jack-2, courses=[Course(name=English-2)])] |
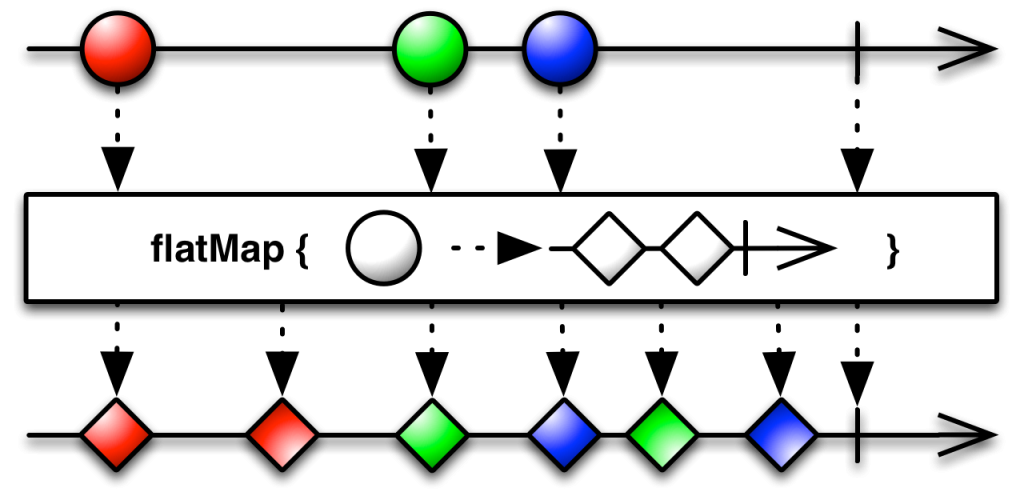

flatMap将一个发送事件的上游Observable变换为多个发送事件的Observables,然后将它们发射的事件合并后放进一个单独的Observable里。需要注意的是, flatMap并不保证事件的顺序,也就是说转换之后的Observables的顺序不必与转换之前的序列的顺序一致。
与flatMap对应的方法是contactMap,后者能够保证最终输出的顺序与上游发送的顺序一致。
Both methods look pretty much the same, but there is a difference: operator usage when merging the final results.
The flatMap() method creates a new Observable by applying a function that you supply to each item emitted by the original Observable, where that function is itself an Observable that emits items, and then merges the results of that function applied to every item emitted by the original Observable, emitting these merged results.
Note that flatMap() may interleave the items emitted by the Observables that result from transforming the items emitted by the source Observable.
If it is important that these items not be interleaved, you can instead use the similar concatMap() method.
FlatMap对这些Observables发射的数据做的是合并(merge)操作,因此它们可能是交错的。也就说,传入的顺序可能跟出来的顺序不一样。如果要保证顺的的话,可以使用concatMap。
flatMapIterable
switchMap
Example 1
1 | val dataManager = DataManager() |
1 | origin = [Student(name=Jack-0, courses=[Course(name=English-0)]), Student(name=Jack-1, courses=[Course(name=English-1)]), Student(name=Jack-2, courses=[Course(name=English-2)])] |
If just return a normal observable on switchMap(), example:
Example 1
1 | Observable.fromIterable(queryOrigin) |
1 | origin = [Student(name=Jack-0, courses=[Course(name=English-0)]), Student(name=Jack-1, courses=[Course(name=English-1)]), Student(name=Jack-2, courses=[Course(name=English-2)])] |
cast
Example 1
1 | Observable.just(1, 2, 3) |
scan
apply a function to each item emitted by an Observable, sequentially, and emit each successive value
Example 1
1 | val dataManager = DataManager() |
1 | --------------------- |
buffer
periodically gather items from an Observable into bundles and emit these bundles rather than emitting the items one at a time
Example 1
1 | Observable.just(1, 2, 3, 4, 5, 6, 7, 8) |
windows
periodically subdivide items from an Observable into Observable windows and emit these windows rather than emitting the items one at a time
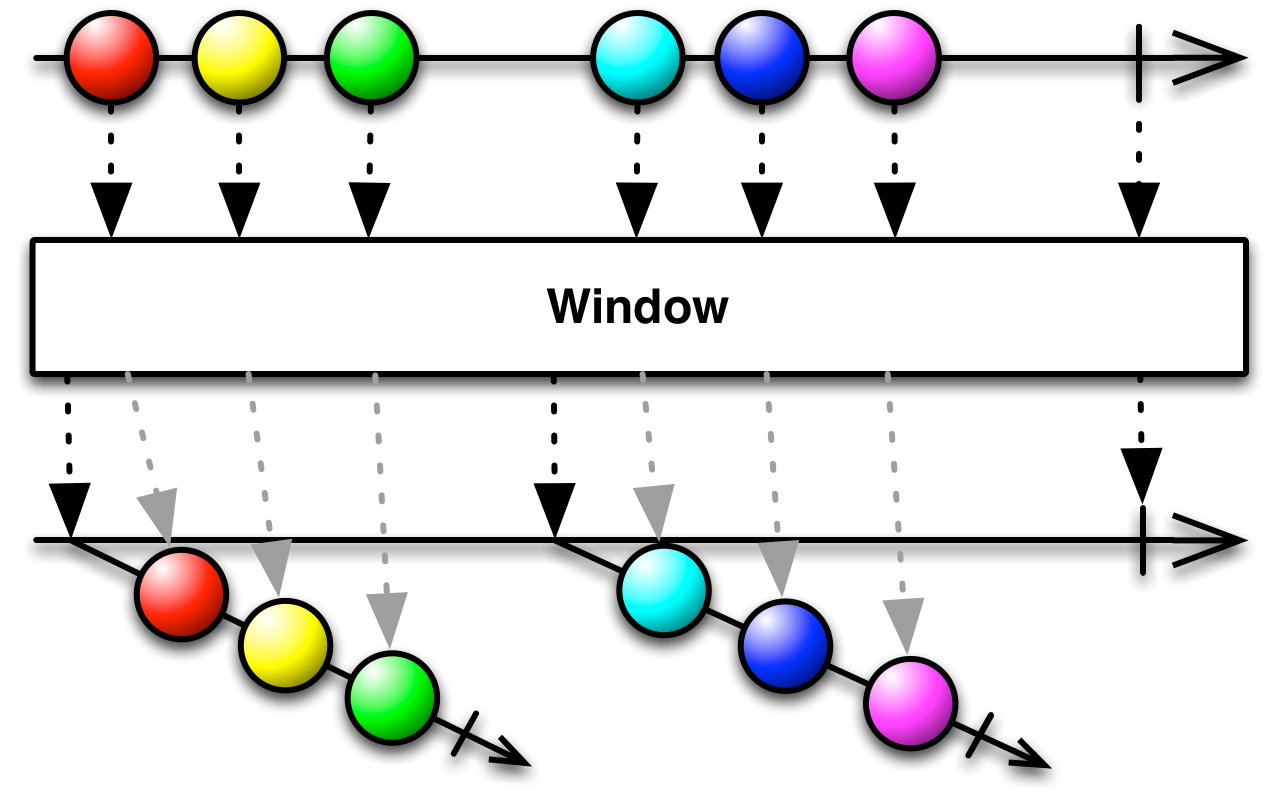
Window is similar to Buffer, but rather than emitting packets of items from the source Observable, it emits Observables, each one of which emits a subset of items from the source Observable and then terminates with an onCompleted notification.
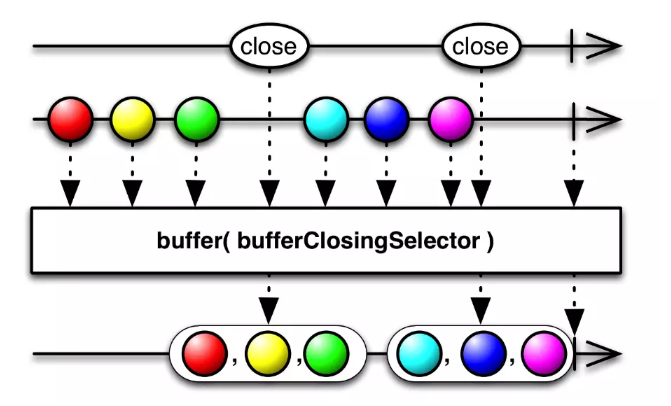
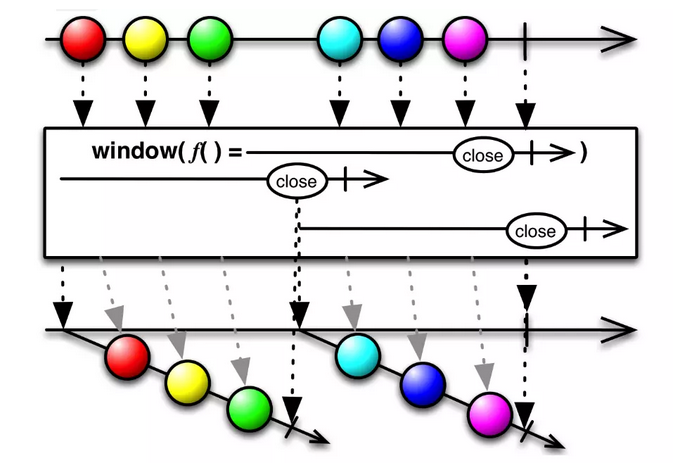
Window和Buffer类似,但不是发射来自原始Observable的数据包,它发射的是Observable,这些Observables中的每一个都发射原始Observable数据的一个子集,最后发射一个onCompleted通知。
以下面的程序为例,这里我们首先生成了一个由10个数字组成的整数序列,然后使用window函数将它们每3个作为一组,每组会返回一个对应的Observable对象。
这里我们对该返回的结果进行订阅并进行消费,因为10个数字,所以会被分成4个组,每个对应一个Observable:
Example 1
1 | Observable.range(1, 10).window(3) |
1 | 901506536 : 1 |
groupBy
divide an Observable into a set of Observables that each emit a different group of items from the original Observable, organized by key
groupBy用于分组元素,它可以被用来根据指定的条件将元素分成若干组。
Example 1
1 | Observable.concat( |
1 | key 1 -> value 1 |
Math
average
count
max
min
reduce
apply a function to each item emitted by an Observable, sequentially, and emit the final value
Reference
- http://reactivex.io/documentation/operators.html
- https://maxwell-nc.github.io/android/rxjava2-6.html
- https://medium.com/mindorks/rxjava-operator-map-vs-flatmap-427c09678784
- https://fernandocejas.com/2015/01/11/rxjava-observable-tranformation-concatmap-vs-flatmap/
- https://my.oschina.net/u/2277632/blog/2986715
- https://juejin.im/post/5b72f76551882561354462dd